When AmiBroker is fed with the data, say 1-minute data, it can create all other time intervals by compressing source data on-the-fly. So if you display say 13-minute chart, AmiBroker takes source 1-minute data and builds 13-minute blocks of data to create 13-minute bars. For this process to work correctly, source data need to have timestamps that point to the START of each bar interval. So with 1-minute data, the bar that has a timestamp 9:30:00 is supposed to cover trades from the period of 9:30:00.000 upto 9:30:59.999. All our data plugins provide data in that format.
Now, provided that we have say 1-minute data, AmiBroker can compress data to any other N-minute interval. When doing so, it can assign timestamps to compressed bars in different ways. This can be controlled through Tools->Preferences->Intraday dialog.
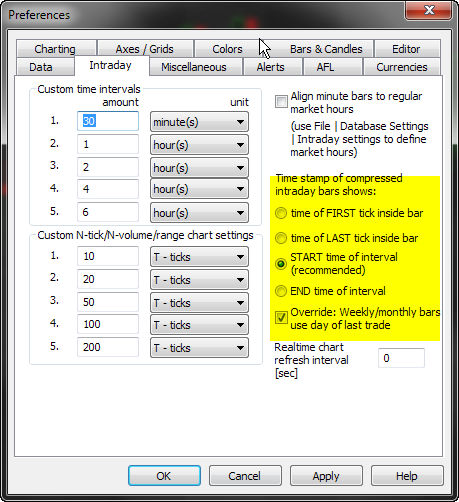
Let us check it on an example of a 5-minute bar based on input 1-minute quotes for e-mini contract.
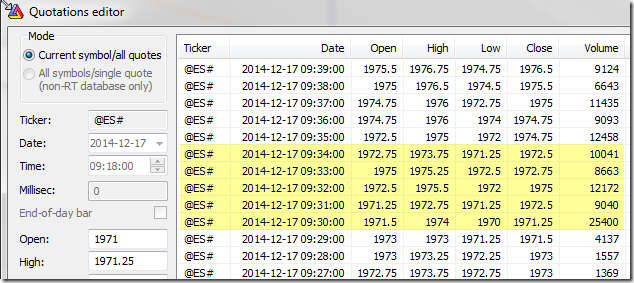
As explained in the manual (http://www.amibroker.com/guide/w_preferences.html) – there are four choices available:
- Time of FIRST tick inside bar – when selected the bar gets the time stamp of the very first trade inside given time slot (bar). With this choice the bar will be stamped with 9:30:00 because this is the first tick (quote) available within that 5-min period
- Time of the LAST tick inside bar – when selected the bar gets the time stamp of the very last trade inside given time slot (bar). In this case the bar will be stamped with 9:34:00 because this is the last quote available within that 5-min period
- START time of the interval – when selected the bar is time-stamped with start time of the time slot (bar). The bar will be stamped with 9:30:00 because that is a beginning of the selected time period.
NOTE: This is recommended and the default setting as it provides consistency with how source bars are timestamped. It should not be changed unless you have really good reason to do so. - END time of the interval – when selected the bar is time-stamped with start time of the time slot (bar). The bar will be stamped with 9:34:59 timestamp, because that’s the very end of this 5-min period.
There is also an additional setting available (Override: Weekly/monthly bars use day of last trade), which allow to modify the behaviour in case of Weekly/Monhtly bars, no matter what is the main setting we use. This allows us to e.g. use START time of interval to identify intraday quotes, however – on a weekly chart display e.g. Wednesday date (if that is most recent day in current week) or Friday date for complete weeks.
We need to remember that the timestamps identify the whole bar and all trades within that bar, so if we use START time of interval for time-stamping, in the backtest use Close array for as BuyPrice and 5-minute periodicity, then in our report we will see:

So, we see the time 9:30:00, but this bar refers to trading activity from period 9:30:00-9:34:59 and the actual price is read from the tick being the Close of the whole 5-minute period (at 9:34:00 in the table above).
For the same reason – when we use weekly data for backtesting, we trade at Open, but for time-stamps we use Override box (so weekly bars are stamped with the data of the last day within given week) – then in the report we will see e.g. Friday dates because of the fact that we use such approach to time-stamp bars. This does not really mean that trade happened on Friday, but only that we use Friday date to identify the whole Monday-to-Friday week.